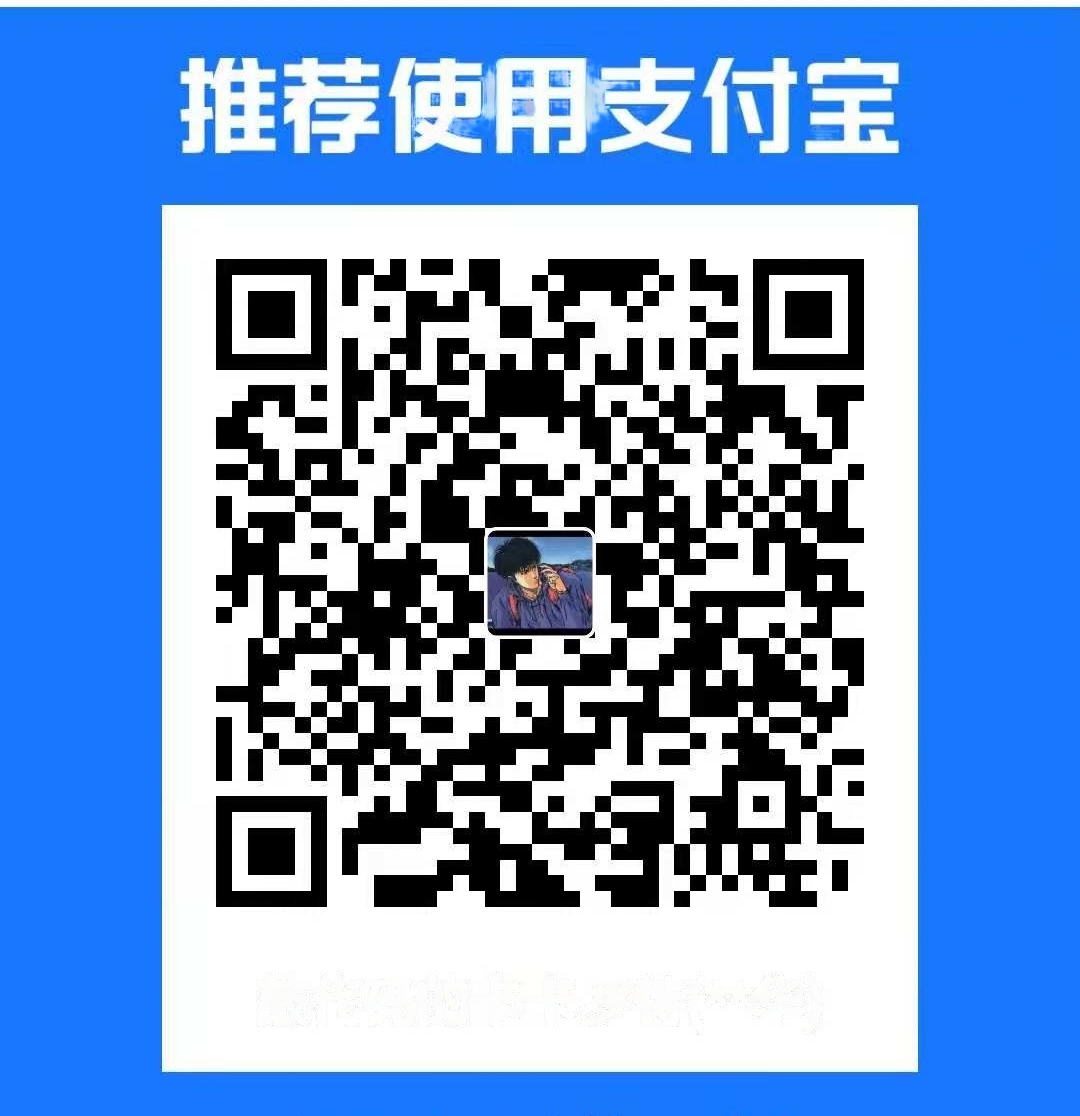
02-HttpClient工具类
httpClient实现
pom.xml
xml
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.14</version>
</dependency>
get请求
java
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.conn.ssl.NoopHostnameVerifier;
import org.apache.http.conn.ssl.SSLConnectionSocketFactory;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.ssl.SSLContextBuilder;
import org.apache.http.util.EntityUtils;
import javax.net.ssl.SSLContext;
import java.security.KeyManagementException;
import java.security.KeyStoreException;
import java.security.NoSuchAlgorithmException;
public class ApacheHttpClientExample {
public static CloseableHttpClient createHttpClient() throws NoSuchAlgorithmException, KeyStoreException, KeyManagementException {
// 安装信任所有证书的SSLContext
SSLContext sslContext = SSLContextBuilder.create()
.loadTrustMaterial(null, (chain, authType) -> true)
.build();
// 创建一个允许所有主机名的HostnameVerifier
SSLConnectionSocketFactory sslsf = new SSLConnectionSocketFactory(sslContext, NoopHostnameVerifier.INSTANCE);
// 创建并返回HttpClient实例
return HttpClients.custom()
.setSSLSocketFactory(sslsf)
.build();
}
public static void main(String[] args) {
String url = "http://example.com/api"; // 替换为实际请求的URL
try {
CloseableHttpClient httpClient = createHttpClient(); //HttpClients.createDefault();
// 添加请求参数
String param1 = "value1";
String param2 = "value2";
String fullUrl = url + "?param1=" + param1 + "¶m2=" + param2;
HttpGet get = new HttpGet(fullUrl);
// 添加请求头
get.setHeader("Authorization", "Bearer your_token"); // 替换为实际Token
// 添加请求Cookie
get.setHeader("Cookie", "name=value");
// 执行请求
HttpResponse response = httpClient.execute(get);
// 打印响应结果
String responseBody = EntityUtils.toString(response.getEntity());
System.out.println("Response Body: " + responseBody);
// 打印返回头信息
System.out.println("Response Headers:");
for (org.apache.http.Header header : response.getAllHeaders()) {
System.out.println(header.getName() + ": " + header.getValue());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
post请求
java
import org.apache.http.HttpEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.conn.ssl.NoopHostnameVerifier;
import org.apache.http.conn.ssl.SSLConnectionSocketFactory;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.ssl.SSLContextBuilder;
import org.apache.http.util.EntityUtils;
import javax.net.ssl.SSLContext;
import java.security.KeyManagementException;
import java.security.KeyStoreException;
import java.security.NoSuchAlgorithmException;
public class ApacheHttpClientExample {
public static CloseableHttpClient createHttpClient() throws NoSuchAlgorithmException, KeyStoreException, KeyManagementException {
// 安装信任所有证书的SSLContext
SSLContext sslContext = SSLContextBuilder.create()
.loadTrustMaterial(null, (chain, authType) -> true)
.build();
// 创建一个允许所有主机名的HostnameVerifier
SSLConnectionSocketFactory sslsf = new SSLConnectionSocketFactory(sslContext, NoopHostnameVerifier.INSTANCE);
// 创建并返回HttpClient实例
return HttpClients.custom()
.setSSLSocketFactory(sslsf)
.build();
}
public static void main(String[] args) {
String url = "http://example.com/api"; // 替换为实际的 URL
try {
CloseableHttpClient httpClient = createHttpClient(); //HttpClients.createDefault();
HttpPost post = new HttpPost(url);
// 设置请求头
post.setHeader("Content-Type", "application/json");
post.setHeader("Authorization", "Bearer your_token"); // 替换为实际的 token
// 设置 Cookie
post.setHeader("Cookie", "key=value"); // 替换为实际的 cookie
// 设置请求参数(JSON 格式)
String json = "{\"param1\":\"value1\",\"param2\":\"value2\"}"; // 替换为实际的参数
post.setEntity(new StringEntity(json));
// 执行请求
CloseableHttpResponse response = httpClient.execute(post);
try {
HttpEntity responseEntity = response.getEntity();
// 打印响应结果
String result = EntityUtils.toString(responseEntity);
System.out.println("Response: " + result);
// 打印返回头信息
System.out.println("Response Headers:");
for (org.apache.http.Header header : response.getAllHeaders()) {
System.out.println(header.getName() + ": " + header.getValue());
}
} finally {
response.close();
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
工具类
java
import lombok.extern.slf4j.Slf4j;
import org.apache.http.Consts;
import org.apache.http.HttpEntity;
import org.apache.http.NameValuePair;
import org.apache.http.ParseException;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.config.RequestConfig;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.conn.ssl.SSLConnectionSocketFactory;
import org.apache.http.conn.ssl.SSLContexts;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.impl.conn.PoolingHttpClientConnectionManager;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.util.EntityUtils;
import javax.net.ssl.SSLContext;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.security.KeyStore;
import java.security.NoSuchAlgorithmException;
import java.security.cert.CertificateException;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.UUID;
/**
* httpClient 请求工具类
*/
@Slf4j
public class HttpClientUtil {
private static PoolingHttpClientConnectionManager cm;
static {
cm = new PoolingHttpClientConnectionManager();
//设置最大连接数
cm.setMaxTotal(100);
//设置每个主机的最大连接数
cm.setDefaultMaxPerRoute(10);
}
//设置请求信息
private static RequestConfig getConfig() {
RequestConfig config = RequestConfig.custom()
.setConnectTimeout(1000) //创建连接的最长时间
.setConnectionRequestTimeout(500) // 获取连接的最长时间
.setSocketTimeout(10000) //数据传输的最长时间
.build();
return config;
}
/**
* 根据请求地址下载页面数据
*
* @param url
* @return 页面数据
*/
public static String doGetHtml(String url) {
//获取HttpClient对象
CloseableHttpClient httpClient = HttpClients.custom().setConnectionManager(cm).build();
//创建httpGet请求对象,设置url地址
HttpGet httpGet = new HttpGet(url);
//设置请求信息
httpGet.setConfig(getConfig());
CloseableHttpResponse response = null;
try {
//使用HttpClient发起请求,获取响应
response = httpClient.execute(httpGet);
//解析响应,返回结果
if (response.getStatusLine().getStatusCode() == 200) {
//判断响应体Entity是否不为空,如果不为空就可以使用EntityUtils
if (response.getEntity() != null) {
String content = EntityUtils.toString(response.getEntity(), "utf8");
return content;
}
}
} catch (IOException e) {
e.printStackTrace();
} finally {
//关闭response
if (response != null) {
try {
response.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
//返回空串
return "";
}
/**
* 下载图片
*
* @param url 需要下载的url
* @param targetPath 下载的文件夹路径
* @return 图片名称
*/
public static String doGetImage(String url, String targetPath) {
CloseableHttpResponse response = null;
try {
//获取HttpClient对象
CloseableHttpClient httpClient = HttpClients.custom()
.setConnectionManager(cm).build();
//创建httpGet请求对象,设置url地址
HttpGet httpGet = new HttpGet(url);
//设置请求信息
httpGet.setConfig(getConfig());
//使用HttpClient发起请求,获取响应
response = httpClient.execute(httpGet);
//解析响应,返回结果
if (response.getStatusLine().getStatusCode() == 200) {
//判断响应体Entity是否不为空
if (response.getEntity() != null) {
//下载图片
//获取图片的后缀
String extName = url.substring(url.lastIndexOf("."));
//创建图片名,重命名图片
String picName = UUID.randomUUID().toString() + extName;
if (!targetPath.endsWith(String.valueOf(File.separatorChar))) {
targetPath += File.separatorChar;
}
String fullPath = targetPath + picName;
//下载图片
//声明OutPutStream
OutputStream outputStream = new FileOutputStream(new File(fullPath));
response.getEntity().writeTo(outputStream);
//返回图片名称
return fullPath;
}
}
} catch (Exception e) {
e.printStackTrace();
} finally {
//关闭response
if (response != null) {
try {
response.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
//如果下载失败,返回空串
return "";
}
/**
* 以jsonString形式发送HttpPost的Json请求,String形式返回响应结果
*
* @param url
* @param jsonString
* @return
*/
public static String sendPostJsonStr(String url, String jsonString) throws IOException {
if (jsonString == null || jsonString.isEmpty()) {
return post(url);
}
String resp = "";
StringEntity entityStr = new StringEntity(jsonString,
ContentType.create("text/plain", "UTF-8"));
CloseableHttpClient httpClient = HttpClients.custom().setConnectionManager(cm).build();
HttpPost httpPost = new HttpPost(url);
httpPost.setEntity(entityStr);
CloseableHttpResponse response = null;
try {
response = httpClient.execute(httpPost);
HttpEntity entity = response.getEntity();
resp = EntityUtils.toString(entity, "UTF-8");
EntityUtils.consume(entity);
} catch (ClientProtocolException e) {
log.error(e.getMessage());
} catch (IOException e) {
log.error(e.getMessage());
} finally {
if (response != null) {
try {
response.close();
} catch (IOException e) {
log.error(e.getMessage());
}
}
}
if (resp == null || resp.equals("")) {
return "";
}
return resp;
}
public static String sendPostMap(String url, Map<String, String> paramMap) throws IOException {
if (paramMap == null || paramMap.isEmpty()) {
return post(url);
}
List<NameValuePair> nvps = new LinkedList<NameValuePair>();
for (String key : paramMap.keySet()) {
nvps.add(new BasicNameValuePair(key, paramMap.get(key))); // 参数
}
String resp = "";
CloseableHttpClient httpClient = HttpClients.custom().setConnectionManager(cm).build();
HttpPost httpPost = new HttpPost(url);
httpPost.setEntity(new UrlEncodedFormEntity(nvps, Consts.UTF_8)); // 设置参数
CloseableHttpResponse response = null;
try {
response = httpClient.execute(httpPost);
HttpEntity entity = response.getEntity();
resp = EntityUtils.toString(entity, "UTF-8");
EntityUtils.consume(entity);
} catch (ClientProtocolException e) {
log.error(e.getMessage());
} catch (IOException e) {
log.error(e.getMessage());
} finally {
if (response != null) {
try {
response.close();
} catch (IOException e) {
log.error(e.getMessage());
}
}
}
if (resp == null || resp.equals("")) {
return "";
}
return resp;
}
/**
* 发送不带参数的HttpPost请求
*
* @param url
* @return
*/
public static String post(String url) throws IOException {
// 1.获得一个httpclient对象
CloseableHttpClient httpclient = HttpClients.custom().setConnectionManager(cm).build();
// 2.生成一个post请求
HttpPost httppost = new HttpPost(url);
CloseableHttpResponse response = null;
try {
// 3.执行get请求并返回结果
response = httpclient.execute(httppost);
} catch (IOException e) {
log.error(e.getMessage());
}
// 4.处理结果,这里将结果返回为字符串
HttpEntity entity = response.getEntity();
String result = null;
try {
result = EntityUtils.toString(entity);
} catch (ParseException | IOException e) {
log.error(e.getMessage());
}
return result;
}
/**
* 带参的get请求
*
* @param url
* @param param
* @return
* @throws ClientProtocolException
* @throws IOException
*/
public static String get(String url, Map<String, String> param) {
if (param != null) {
StringBuilder url_ = new StringBuilder(url);
boolean isFirst = true;
for (String key : param.keySet()) {
if (isFirst) {
url_.append("?");
} else {
url_.append("&");
isFirst = false;
}
url_.append(key).append("=").append(param.get(key));
}
url = url_.toString();
}
// 1.获得一个httpclient对象
CloseableHttpClient httpclient = HttpClients.custom().setConnectionManager(cm).build();
// 2.生成一个get请求
HttpGet httpGet = new HttpGet(url);
CloseableHttpResponse response = null;
try {
// 3.执行get请求并返回结果
response = httpclient.execute(httpGet);
} catch (IOException e) {
log.error(e.getMessage());
}
// 4.处理结果,这里将结果返回为字符串
HttpEntity entity = response.getEntity();
String result = null;
try {
result = EntityUtils.toString(entity);
} catch (ParseException | IOException e) {
log.error(e.getMessage());
}
return result;
}
/**
* 不带参的get请求
*
* @param url
* @return
* @throws ClientProtocolException
* @throws IOException
*/
public static String get(String url) throws ClientProtocolException, IOException {
return get(url, null);
}
/**
* 发送xml参数的请求,常用于微信开发。
*
* @param url
* @param xmlParam
* @return
* @throws IOException
*/
public static String sendPostXml(String url, String xmlParam) throws IOException {
// 1.获得一个httpclient对象
CloseableHttpClient httpclient = HttpClients.custom().setConnectionManager(cm).build();
// 2.生成一个post请求
HttpPost httppost = new HttpPost(url);
if (xmlParam != null) {
httppost.setEntity(new StringEntity(xmlParam, Consts.UTF_8));
}
CloseableHttpResponse response = null;
try {
// 3.执行get请求并返回结果
response = httpclient.execute(httppost);
} catch (IOException e) {
log.error(e.getMessage());
}
// 4.处理结果,这里将结果返回为字符串
HttpEntity entity = response.getEntity();
String result = null;
try {
result = EntityUtils.toString(entity, Consts.UTF_8);
} catch (ParseException | IOException e) {
log.error(e.getMessage());
}
return result;
}
/**
* 发送带ssl的post请求,专门用户微信 企业到零钱
*
* @param url
* @param xmlParam
* @param path
* @param mchid
* @return
* @throws Exception
*/
public static String sendSSlPostXml(String url, String xmlParam, String path, String mchid) throws Exception {
// 1.获得一个httpclient对象
CloseableHttpClient httpclient = HttpClients.custom()
.setConnectionManager(cm)
.setSSLSocketFactory(loadSsl(path, mchid))
.build();
// 2.生成一个post请求
HttpPost httppost = new HttpPost(url);
if (xmlParam != null) {
httppost.setEntity(new StringEntity(xmlParam, Consts.UTF_8));
}
httppost.addHeader("Content-Type", "text/xml");
CloseableHttpResponse response = null;
try {
// 3.执行get请求并返回结果
response = httpclient.execute(httppost);
} catch (IOException e) {
log.error(e.getMessage());
}
// 4.处理结果,这里将结果返回为字符串
HttpEntity entity = response.getEntity();
String result = null;
try {
result = EntityUtils.toString(entity, Consts.UTF_8);
} catch (ParseException | IOException e) {
log.error(e.getMessage());
}
return result;
}
/**
* 加载微信ssl证书
*
* @param path 证书路径
* @param mchid 商户mchid
* @return
* @throws Exception
*/
private static SSLConnectionSocketFactory loadSsl(String path, String mchid) throws Exception {
KeyStore keyStore = KeyStore.getInstance("PKCS12");
//加载本地的证书进行https加密传输
FileInputStream instream = new FileInputStream(new File(path));
try {
//加载证书密码,默认为商户密钥
keyStore.load(instream, mchid.toCharArray());
} catch (IOException e) {
e.printStackTrace();
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
} catch (CertificateException e) {
e.printStackTrace();
} finally {
instream.close();
}
// Trust own CA and all self-signed certs
SSLContext sslcontext = SSLContexts.custom().loadKeyMaterial(keyStore, mchid.toCharArray()).build();
// Allow TLSv1 protocol only
SSLConnectionSocketFactory sslsf = new SSLConnectionSocketFactory(sslcontext,
new String[]{"TLSv1"}, null,
SSLConnectionSocketFactory.BROWSER_COMPATIBLE_HOSTNAME_VERIFIER);
return sslsf;
}
}
测试
java
import ch.qos.logback.classic.Level;
import ch.qos.logback.classic.Logger;
import ch.qos.logback.classic.LoggerContext;
import org.junit.Test;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.util.List;
public class Test3 {
static {
LoggerContext loggerContext = (LoggerContext) LoggerFactory.getILoggerFactory();
List<Logger> loggerList = loggerContext.getLoggerList();
loggerList.forEach(logger -> {
logger.setLevel(Level.INFO);
});
}
@Test
public void test() throws Exception {
String url = "https://www.baidu.com/";
String html = HttpClientUtil.doGetHtml(url);
System.out.println(html);
}
@Test
public void test2() throws Exception {
String url = "https://img2020.cnblogs.com/blog/1149398/202109/1149398-20210928171248706-226007316.gif";
// String url = "https://blog.share888.top/logo.png"; // 这个下载报错 ssl的问题
String targetPath = "C:\\Users\\Think\\Desktop";
String html = HttpClientUtil.doGetImage(url,targetPath);
System.out.println(html);
}
@Test
public void test3() throws Exception {
System.out.println(File.separator);
System.out.println(File.separatorChar);
System.out.println(File.pathSeparator);
}
}
okhttp
依赖
xml
<dependency>
<groupId>com.squareup.okhttp3</groupId>
<artifactId>okhttp</artifactId>
<version>4.9.3</version> <!-- 请根据需要选择版本 -->
</dependency>
get请求
java
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
import okhttp3.HttpUrl;
import java.io.IOException;
public class OkHttpExample {
public static void main(String[] args) {
OkHttpClient client = new OkHttpClient();
// 添加请求参数
String url = "http://example.com/api"; // 替换为实际请求的URL
HttpUrl urlWithParams = HttpUrl.parse(url).newBuilder()
.addQueryParameter("param1", "value1")
.addQueryParameter("param2", "value2")
.build();
// 构建请求
Request request = new Request.Builder()
.url(urlWithParams)
.addHeader("Authorization", "Bearer your_token") // 替换为实际Token
.addHeader("Cookie", "name=value")
.build();
try (Response response = client.newCall(request).execute()) {
// 打印响应结果
if (response.isSuccessful()) {
System.out.println("Response Body: " + response.body().string());
} else {
System.out.println("Unexpected code " + response);
}
// 打印返回头信息
System.out.println("Response Headers:");
for (String name : response.headers().names()) {
System.out.println(name + ": " + response.header(name));
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
post请求
java
import okhttp3.*;
import java.io.IOException;
public class OkHttpExample {
public static void main(String[] args) {
String url = "http://example.com/api"; // 替换为实际的 URL
OkHttpClient client = new OkHttpClient();
// 构建请求体
MediaType JSON = MediaType.get("application/json; charset=utf-8");
String json = "{\"param1\":\"value1\",\"param2\":\"value2\"}"; // 替换为实际的参数
RequestBody body = RequestBody.create(json, JSON);
// 构建请求
Request request = new Request.Builder()
.url(url)
.post(body)
.addHeader("Authorization", "Bearer your_token") // 替换为实际的 token
.addHeader("Cookie", "key=value") // 替换为实际的 cookie
.build();
try (Response response = client.newCall(request).execute()) {
// 打印响应结果
if (!response.isSuccessful()) {
throw new IOException("Unexpected code " + response);
}
System.out.println("Response: " + response.body().string());
// 打印返回头信息
System.out.println("Response Headers:");
for (String name : response.headers().names()) {
System.out.println(name + ": " + response.header(name));
}
} catch (IOException e) {
e.printStackTrace();
}
}
}