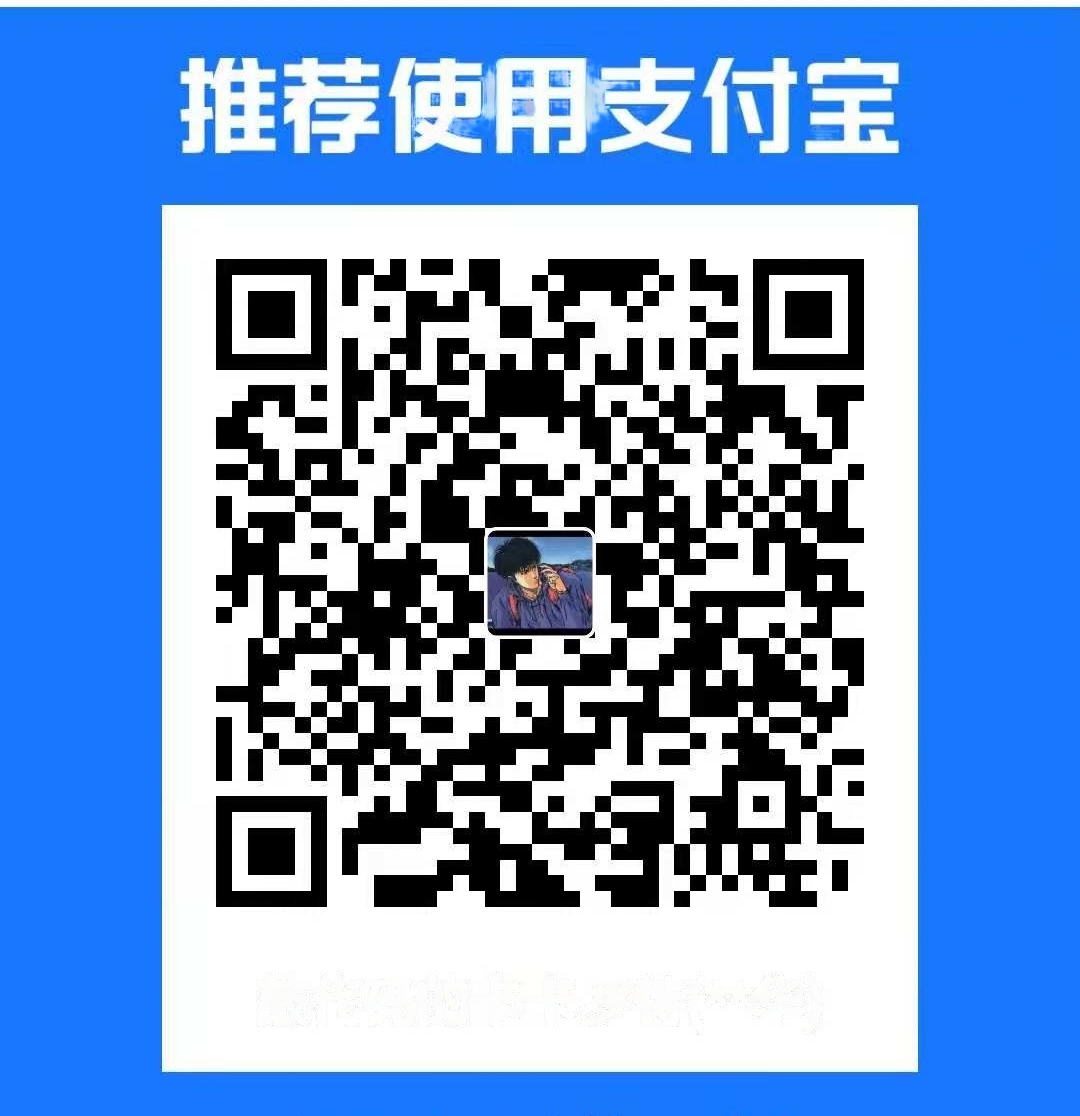
autojs常用api
常见控件的类名
js
android.widget.TextView 文本控件
android.widget.ImageView 图片控件
android.widget.Button 按钮控件
android.widget.EditText 输入框控件
android.widget.AbsListView 列表控件
android.widget.LinearLayout 线性布局
android.widget.FrameLayout 帧布局
android.widget.RelativeLayout 相对布局
android.widget.RelativeLayout 相对布局
android.support.v7.widget.RecyclerView 通常也是列表控件
查找控件
js
className("android.widget.FrameLayout").desc("红包签到").find()
className("android.widget.FrameLayout").desc("红包签到").find().size()
控件属性
js
/**
* 打印控件信息 可以传递 数组或者对象
* @param {控件} node
*/
function PrintNodeInfo(ele) {
log("开始打印控件--------------->");
if (ele && Array.isArray(ele)) {
for (let i = 0; i < ele.length; i++) {
const node = ele[i];
PrintNodeInfoByNode(node);
console.log("\r\n");
}
} else if (ele) {
PrintNodeInfoByNode(ele);
}
log("控件打印完毕--------------->");
}
function PrintNodeInfoByNode(node) {
log("元素的类名: " + node.className());
log("元素的文本: " + node.text());
log("元素的深度: " + node.depth());
log("元素的描述: " + node.desc());
log("元素坐标: " + node.bounds());
log("元素是否可以被点击: " + node.clickable());
log("元素是否选中: " + node.checked());
log("元素是否可滑动: " + node.scrollable());
log("元素是否可见: " + node.visibleToUser());
}
坐标
js
bounds().left:长方形左边界的x坐标
bounds().right:长方形右边界的x坐标
bounds().top:长方形上边界的y坐标
bounds().bottom:长方形下边界的y坐标
bounds().centerX():长方形中点x坐标
bounds().centerY():长方形中点y坐标
bounds().width():长方形宽度也就是控件宽度
bounds().height():长方形高度也就是控件高度
click(x,y):坐标(x,y)处执行点击操作 //注意:安卓7以下点击需要root权限且函数为Tap(x,y)
事件相关
退出事件
就是调用exit()
的时候会触发的回调
js
// 监听退出事件
events.on("exit", function () {
console.log("脚本即将退出...");
log("3秒之后关闭程序");
exit();
});
监听按键
js
//启用按键监听
events.observeKey();
//监听音量上键按下
events.onKeyDown("volume_up", function(event){
toast("音量上");
});
//监听音量下键弹起
events.onKeyDown("volume_down", function(event){
toast("音量下");
});
//监听Home键弹起
events.onKeyDown("home", function(event){
toast("Home");
});
//监听菜单键按下
events.onKeyDown("menu", function(event){
toast("菜单键被按下了");
});
滑动
swipe上下滑动和翻页
swipe(x1, y1, x2, y2, duration)
- x1 {number} 滑动的起始坐标的x值
- y1 {number} 滑动的起始坐标的y值
- x2 {number} 滑动的结束坐标的x值
- y2 {number} 滑动的结束坐标的y值
- duration {number} 滑动时长,单位毫秒
js
const h = device.height // 屏幕高
const w = device.width // 屏幕宽
const x1 = (w / 3) * 2 // 横坐标3分之2处
const x2 = (w / 3) * 1 // 横坐标3分之1处
const h1 = (h / 6) * 3 // 纵坐标6分之3处
const h2 = (h / 6) * 5 // 纵坐标6分之5处
const h3 = h / 6 // 纵坐标6分之1处
swipe(x1, h1, x2, h1, 50) // 向左滑
swipe(x2, h1, x1, h1, 50) // 向右滑
swipe(x1, h1, x1, h2, 50) // 向下滑
swipe(x1, h2, x1, h3, 50) // 向上滑
翻页
js
//向上滑动一页
className("android.support.v7.widget.RecyclerView").findOne().scrollForward();
//向下滑动一页
className("android.support.v7.widget.RecyclerView").findOne().scrollBackward();
系统相关
设备信息
屏幕宽高
js
const h = device.height // 屏幕高
const w = device.width // 屏幕宽
// 有时候获取不到 值为0 可以试试下面的写法
console.log(Packages.android.content.res.Resources.getSystem().getDisplayMetrics().widthPixels);
console.log(Packages.android.content.res.Resources.getSystem().getDisplayMetrics().heightPixels);
息屏
js
var success = runtime.accessibilityBridge.getService().performGlobalAction(android.accessibilityservice.AccessibilityService.GLOBAL_ACTION_LOCK_SCREEN)
唤醒屏幕
没有返回值
js
device.wakeUp()
设备长亮
js
// 一直保持屏幕常亮 长亮一个小时,如果时间为null,则一直长亮下去,建议加时间
device.keepScreenOn(3600 * 1000);
//关闭屏幕常亮
device.cancelKeepingAwake()
解锁
device.isScreenOn() 判断设备屏幕是否亮着的。
js
// 如果是滑动解锁
if (device.isScreenOn() == false) {
device.wakeUp();
sleep(500); // 等待屏幕亮起
// 假设滑动解锁需要从底部滑动到顶部
var x1 = device.width / 2;
var y1 = (device.height * 3) / 4;
var y2 = (device.height * 1) / 4;
swipe(x1, y1, x1, y2, 500); // 滑动操作
sleep(1000); // 等待解锁反应
}
// 如果是PIN码或密码解锁,可以模拟输入
if (device.isScreenOn() == false) {
device.wakeUp();
sleep(500); // 等待屏幕亮起
// 输入PIN口令, 例如1234
setText(0, "1"); // 输入1
setText(1, "2"); // 输入2
setText(2, "3"); // 输入3
setText(3, "4"); // 输入4
// 提交 PIN(根据具体设备,可能需要点击确认按钮)
}
设备信息
js
/*把媒体音量调到最大*/
device.setMusicVolume(device.getMusicMaxVolume());
/*来电,通知铃声设置最大*/
device.setNotificationVolume(device.getNotificationMaxVolume());
打开软件
js
toastLog("脚本开始");
//检查无障碍服务是否启动,若没有则跳转到无障碍服务启动界面,并等待;当服务启动后脚本继续运行
auto.waitFor();
//打开手机App
launchApp("淘宝");
toastLog("执行完了");
exit();
权限相关
请求悬浮窗权限
js
// 请求悬浮窗权限
if (!floaty.checkPermission()) {
floaty.requestPermission();
}
弹框相关
悬浮框
js
var window = floaty.window(
<linear>
<text text="这是一个悬浮窗" />
</linear>
);
window.setPosition(100, 100); // 设置悬浮窗的位置