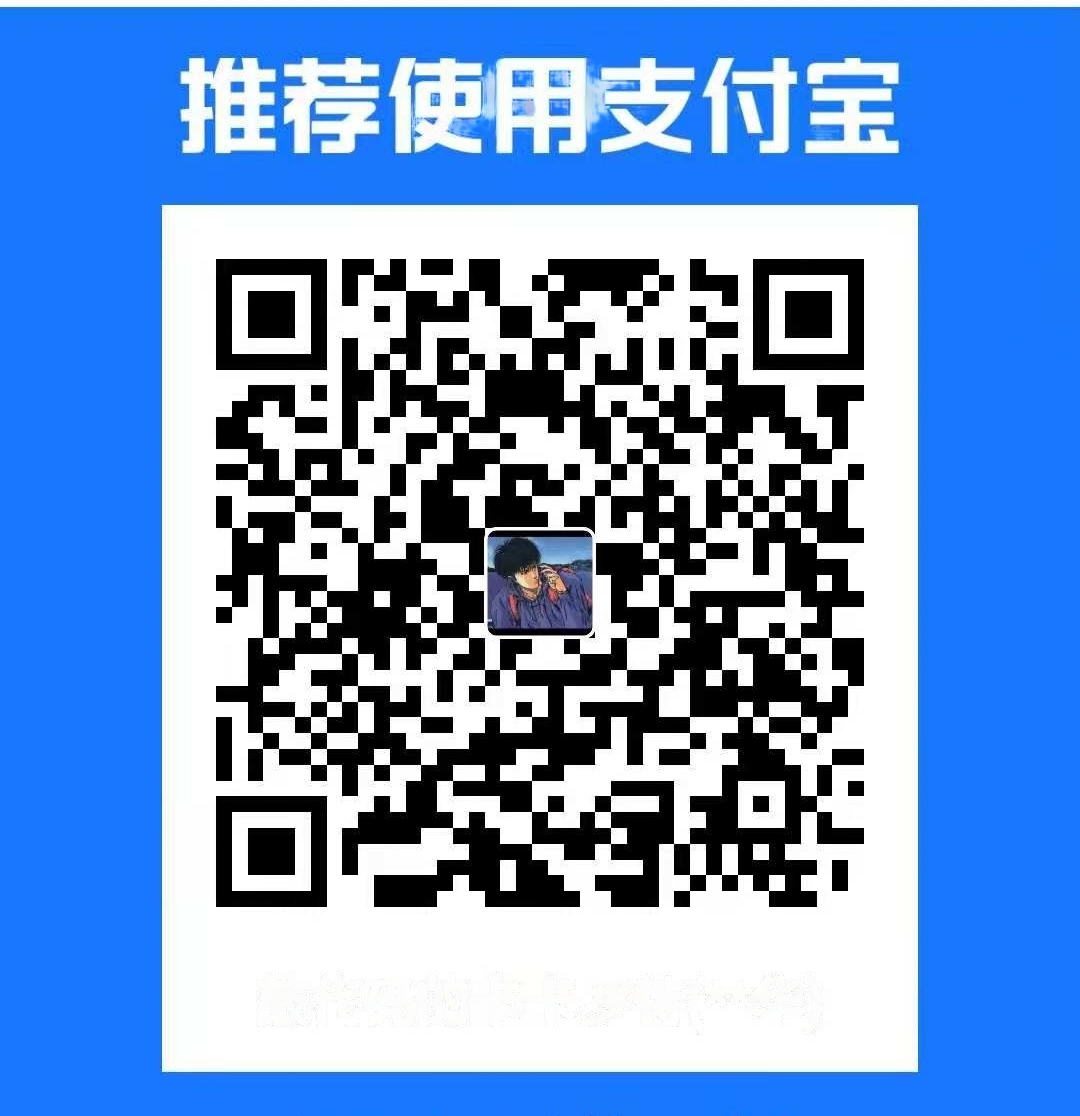
06-ajax请求
fetch请求
原生的 Fetch API
js
//使用URLSearchParams构建查询参数
const params = new URLSearchParams();
params.append('param1', 'value1');
params.append('param2', 'value2');
fetch('https://example.com/api/upload-avatar?' + params.toString(), {
method: 'POST',
headers: {
'Content-Type': 'multipart/form-data',
'token': '123456'
},
body: JSON.stringify({
username: 'admin',
password: '123'
})
})
.then(response => response.json())
.then(data => {
console.log('上传成功', data);
})
.catch(error => {
console.error('上传失败', error);
});
axios的使用
npm安装
shell
npm install axios
yarn add axios
CDN引入
shell
<script src="https://unpkg.com/axios@1.7.7/dist/axios.min.js"></script>
全局设置
js
import axios from 'axios';
// 创建一个axios实例
const instance = axios.create({
baseURL: 'http://your-api-url', // 基础URL
timeout: 1000, // 请求超时时间
// 可以设置默认的headers
headers: {'Content-Type': 'application/json'}
});
get请求
js
axios.get(url, {
params: {
param1: 'value1',
param2: 'value2'
},
headers: {
'Authorization': 'Bearer your-token',
'Content-Type': 'application/json'
},
timeout: 5000,
withCredentials: true, //是否携带跨域请求的凭证。
responseType: 'json',
onUploadProgress: function(progressEvent) {
// 上传进度监听回调函数
},
onDownloadProgress: function(progressEvent) {
// 下载进度监听回调函数
}
}).then(response => {
console.log('Response received:', response.data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
POST请求
带参数,带header的POST请求
js
axios.post(url, data, {
params: {
param1: 'value1',
param2: 'value2'
},
headers: {
'Content-Type': 'application/json'
},
timeout: 5000,
withCredentials: true,
responseType: 'json',
onUploadProgress: function(progressEvent) {
// 上传进度监听回调函数
},
onDownloadProgress: function(progressEvent) {
// 下载进度监听回调函数
}
});
通用请求
js
axios({
method: 'post',
url: 'https://example.com/api/login',
data: {
username: 'admin',
password: '123'
},
params: {
param1: 'value1',
param2: 'value2'
},
headers: {
'Content-Type': 'application/json'
}
}).then(function (response) {
// 处理响应数据
console.log(response.data);
}).catch(function (error) {
// 处理错误情况
console.log(error);
});
自己写的工具类
request.js
工具类1
js
// 引入axios
import axios from 'axios';
import {ElMessage} from "element-plus";
const domain = "http://127.0.0.1/zhongya/api/portal"
// 创建axios实例
const service = axios.create({
baseURL: domain, // api的base_url
timeout: 10000, // 请求超时时间
withCredentials: true, // 是否允许携带cookie跨域
});
// 请求拦截器
service.interceptors.request.use(
config => {
const timeStamp = new Date().getTime()
config.headers['timeStamp'] = timeStamp;
// 在发送请求之前做些什么,例如添加Token
if (localStorage.getItem('token')) {
config.headers['token'] = localStorage.getItem('token');
}
return config;
},
error => {
// 对请求错误做些什么
console.log(error);
return Promise.reject(error);
}
);
// 响应拦截器
service.interceptors.response.use(
response => {
// 对响应数据做点什么
const res = response.data;
if (!res.success) {
if (res.code === 10001){ // 未登录
//应该跳转到登录页面或者其他逻辑
ElMessage.error(res.message)
}else{
ElMessage.error(res.message)
}
}
return res;
},
error => {
// 对响应错误做点什么
console.log('err' + error);
// 可能根据错误码进行不同的错误处理
return Promise.reject(error);
}
);
const request = (option) => {
return new Promise((resolve,reject) => {
service({
url: option.url,
data: option.data || {},
method: option.method || 'GET'
}).then(res=>{
console.log(res)
if (res.success) {
resolve(res)
}
}).catch(err=>{
reject(err)
})
})
}
export default request;
工具类2
js
import axios , { AxiosError, AxiosRequestConfig, AxiosResponse, AxiosInstance } from 'axios'
import { ElMessage } from 'element-plus'
import { useRouter } from 'vue-router'
const baseURL = import.meta.env.VITE_BASE_URL
const service = axios.create({
baseURL: baseURL,
timeout: 5000
})
// 请求前的统一处理
service.interceptors.request.use(
(config) => {
// JWT鉴权处理
if (localStorage.getItem('token')) {
config.headers['token'] = localStorage.getItem('token')
}
return config
},
(error) => {
console.log(error) // for debug
return Promise.reject(error)
}
)
service.interceptors.response.use(
(response) => {
const res = response.data
if (res.code === 200) {
return res
} else {
showError(res)
return Promise.reject(res)
}
},
(error)=> {
console.log(error) // for debug
const badMessage = error.message || error
const code = parseInt(badMessage.toString().replace('Error: Request failed with status code ', ''))
showError({ code, message: badMessage })
return Promise.reject(error)
}
)
// 错误处理
function showError(error) {
// token过期,清除本地数据,并跳转至登录页面
if (error.code === 403) {
// 跳转
const router = useRouter();
router.push({
path:'/login'
})
} else {
ElMessage({
message: error.msg || error.message || '服务异常',
type: 'error',
duration: 3 * 1000
})
}
}
export default service
axios读取文件
vue
<script setup>
import axios from 'axios';
import {ref, onMounted, nextTick, watch} from 'vue';
const str = ref('');
// 读取模板文件
const loadTemplate = async () => {
const response = await axios.get('/xx.txt'); // 记得换成实际的路径
str.value = response.data;
console.log(response.data)
};
// 在组件挂载时加载模板
await loadTemplate();
</script>
原生ajax
js
/**
* post 请求 ,传递json参数
*/
function show() {
let xhr = new XMLHttpRequest();
xhr.open('POST', '/send', true);
xhr.setRequestHeader("Content-Type", "application/json");
let data = {
key1: "value1",
key2: "value2"
};
let jsonData = JSON.stringify(data);
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
console.log(xhr.responseText);
}
};
xhr.send(jsonData);
}
ajax请求
text
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
GET 请求示例
假设你有一个API端点/api/getData,它接受两个查询参数:name和age。以下是如何构造一个包含这些参数的GET请求:
js
// 在AJAX请求中设置Cookie
$.ajax({
url: "/api/getData", //有效接口 https://jsonplaceholder.typicode.com/todos
method: "GET",
data: {
name: 'John Doe', // 参数名: 参数值
age: 30
},
success: function(response) {
console.log(response);
},
error: function(error) {
console.log(error);
}
});
在这个例子中,最终请求的URL会是类似于/api/getData?name=John%20Doe&age=30
的形式
POST 请求示例
现在,假设你有一个API端点/api/saveData,它接收一个JSON对象作为输入。以下是如何构造一个包含数据的POST请求:
js
$.ajax({
url: '/api/saveData', // 请求的目标URL
method: 'POST', // 使用POST方法
contentType: 'application/json', // 设置请求体的内容类型为JSON
data: JSON.stringify({
name: 'John Doe',
age: 30
}), // 将JavaScript对象转换为JSON字符串
success: function(response) {
console.log('成功:', response);
// 在这里处理成功的响应
},
error: function(error) {
console.error('错误:', error);
// 在这里处理错误
}
});
上传文件
html
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.7.1/jquery.min.js"></script>
</head>
<body>
<form id="uploadForm" enctype="multipart/form-data">
<input type="file" name="image" id="imageInput">
<button type="button" id="uploadBtn">上传图片</button>
</form>
<script>
$(document).ready(function() {
$('#uploadBtn').click(function(e) {
e.preventDefault(); // 阻止表单的默认提交行为
var formData = new FormData(); // 创建FormData对象
var fileInput = $('#imageInput')[0]; // 获取文件输入元素
var file = fileInput.files[0]; // 获取选择的文件
if (file) {
formData.append('image', file); // 将文件添加到FormData对象中
$.ajax({
url: '/upload', // 你的服务器端处理上传的URL
type: 'POST', // 请求类型
data: formData, // 发送的数据是FormData对象
contentType: false, // 告诉jQuery不要设置Content-Type请求头
processData: false, // 告诉jQuery不要处理发送的数据
success: function(response) {
// 上传成功后的处理逻辑
console.log('上传成功:', response);
},
error: function(xhr, status, error) {
// 上传失败后的处理逻辑
console.error('上传失败:', error);
}
});
} else {
alert('请选择一个文件');
}
});
});
</script>
</body>
</html>