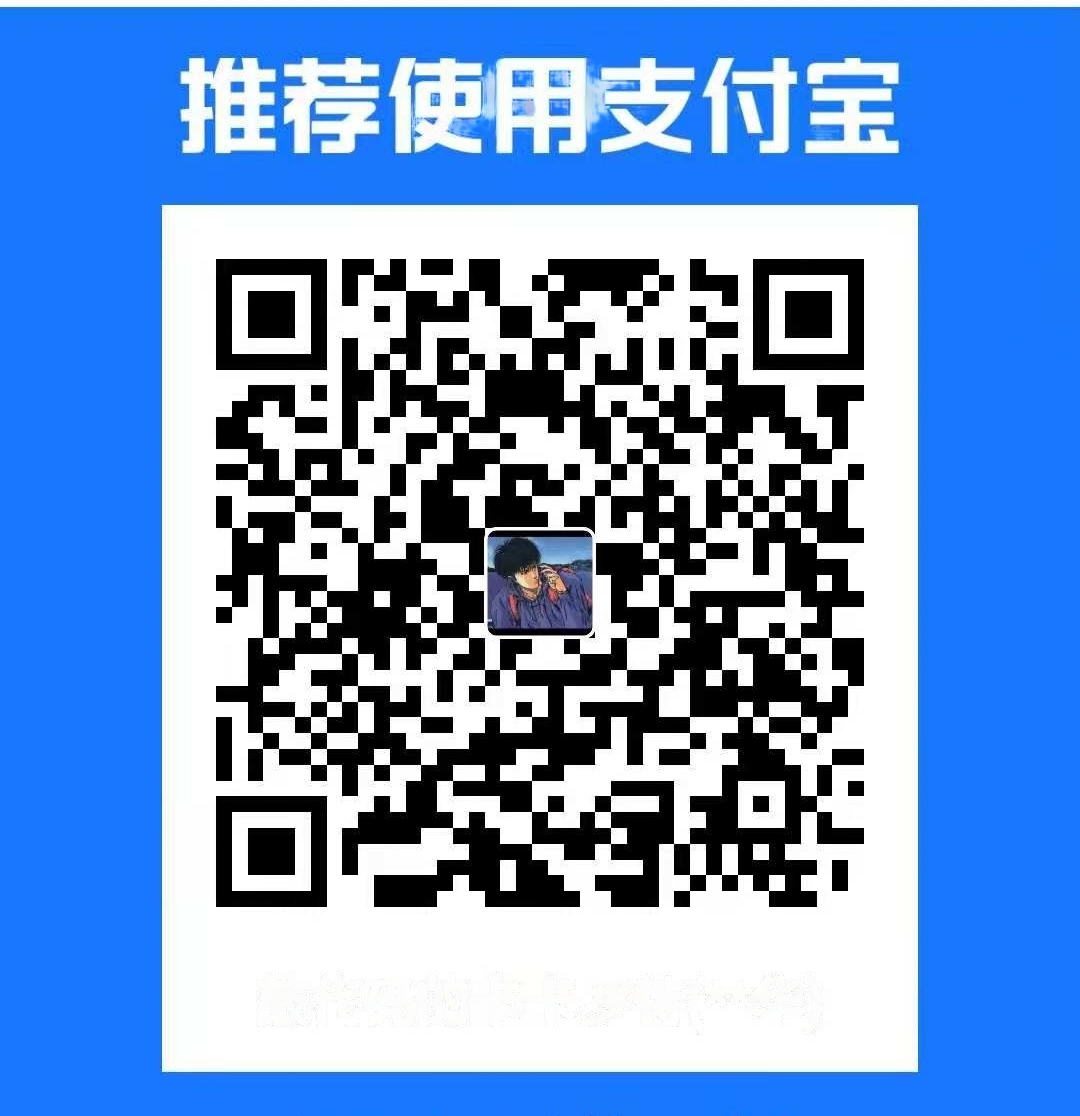
图片相关
a标签导出图片
js
// 如果你想在点击按钮后进行下载,可以创建一个a元素并模拟点击
let a = document.createElement('a');
a.href = imgSrc;
a.download = 'image.jpg'; // 设置下载文件名
document.body.appendChild(a);
a.click();
document.body.removeChild(a); // 清理不再需要的a元素
图片占位服务API
格式:http://via.placeholder.com/宽x高/背景色/字的颜色?text=占位文字
html
<img src="http://via.placeholder.com/200x300/f60/fff?text=HI" alt="">
第二种
html
<img src="https://picsum.photos/200/200" alt="">
第三种
格式:https://dummyimage.com/宽x高/背景色/文字颜色.png&text=显示的文字
html
<img src="https://dummyimage.com/200x100" alt="">
<img src="https://dummyimage.com/200x100/894FC4/FFF.png" alt="">
<img src="https://dummyimage.com/200x100/894FC4/" alt="">
<img src="https://dummyimage.com/200x100/894FC4/FFF.png&text=123" alt="">
如何判断图片全部加载完成
用Promise.all实现
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<body>
<script>
function loadImage(src) {
return new Promise((resolve, reject) => {
const img = new Image();
img.src = src;
img.onload = () => {
resolve(img);
};
img.onerror = () => {
reject(new Error(`Failed to load image at ${src}`));
};
});
}
function loadImages(imageSources) {
const promises = imageSources.map(src => loadImage(src));
return Promise.all(promises);
}
let num = (min,max) => {
return Math.floor(Math.random() * max) + min
}
// 示例使用
const imageUrls = [
`https://picsum.photos/200/${num(100, 300)}?v=` + Math.random(),
`https://picsum.photos/200/${num(100, 300)}?v=` + Math.random()
];
loadImages(imageUrls)
.then(images => {
console.log('所有图片已加载完成', images);
// 这里可以执行后续操作
})
.catch(error => {
console.error('加载图片时发生错误:', error);
});
</script>
</body>
</html>
多个图片打包成zip
shell
npm install jszip
import JSZip from 'jszip';
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>ZIP Download Example</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jszip/3.10.1/jszip.min.js"></script>
</head>
<body>
<button id="download">下载 ZIP 文件</button>
<script>
document.getElementById('download').addEventListener('click', function() {
// 创建一个 JSZip 实例
const zip = new JSZip();
// 假设你有多个图片的 URL
const imageUrls = [
'https://example.com/image1.jpg',
'https://example.com/image2.png',
'https://example.com/image3.gif'
];
const promises = imageUrls.map(url => {
return fetch(url)
.then(response => response.blob())
.then(blob => {
const filename = url.substring(url.lastIndexOf('/') + 1);
zip.file(filename, blob);
});
});
// 等待所有的图片都加载完成
Promise.all(promises).then(() => {
// 生成 ZIP 文件
zip.generateAsync({ type: 'blob' })
.then(content => {
// 创建下载链接
const link = document.createElement('a');
link.href = URL.createObjectURL(content);
link.download = 'images.zip';
document.body.appendChild(link);
link.click();
document.body.removeChild(link);
});
});
});
</script>
</body>
</html>
通过canvas实现图片压缩
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Image Compression</title>
</head>
<body>
<input type="file" id="upload" />
原始图片:
< img id="old" alt="Compressed Image" />
压缩之后的图片:
< img id="result" alt="Compressed Image" />
<script>
document.getElementById('upload').addEventListener('change', function(event) {
const file = event.target.files[0];
if (file) {
const reader = new FileReader();
reader.readAsDataURL(file);
reader.onload = function (e) {
const img = new Image();
img.src = e.target.result;
document.getElementById('old').src = e.target.result;
img.onload = function() {
// 创建一个 canvas 元素并设置宽高
const canvas = document.createElement('canvas');
const ctx = canvas.getContext('2d');
const MAX_WIDTH = 800; // 最大宽度
const MAX_HEIGHT = 800; // 最大高度
let width = img.width;
let height = img.height;
// 计算压缩比例
if (width > height) {
if (width > MAX_WIDTH) {
height *= MAX_WIDTH / width;
width = MAX_WIDTH;
}
} else {
if (height > MAX_HEIGHT) {
width *= MAX_HEIGHT / height;
height = MAX_HEIGHT;
}
}
// 设置 canvas 尺寸
canvas.width = width;
canvas.height = height;
// 绘制图片
ctx.drawImage(img, 0, 0, width, height);
// 导出为压缩后的图片
const dataURL = canvas.toDataURL('image/jpeg', 0.3); // 压缩质量设置为 0.7
document.getElementById('result').src = dataURL;
};
};
}
});
function base64ToFile(base64String, fileName) {
// 将 Base64 字符串分为两部分,分别是 MIME 类型和数据部分
const arr = base64String.split(',');
const mimeType = arr[0].match(/:(.*?);/)[1]; // 提取 MIME 类型
const base64Data = arr[1]; // 提取 Base64 数据
// 解码 Base64 字符串
const byteString = atob(base64Data); // 将 Base64 数据解码为字节字符串
const ab = new ArrayBuffer(byteString.length); // 创建一个 ArrayBuffer
const ia = new Uint8Array(ab); // 将 ArrayBuffer 转换为 Uint8Array
// 将字节字符串中的数据填充到 Uint8Array
for (let i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
// 创建 Blob 对象
const blob = new Blob([ab], { type: mimeType });
// 创建 File 对象并返回
return new File([blob], fileName, { type: mimeType });
}
// 使用示例
const base64Image = 'data:image/png;base64,iVBORw0K...'; // 替换为你的 Base64 字符串
const fileName = 'image.png'; // 文件名
const file = base64ToFile(base64Image, fileName);
console.log(file);
</script>
</body>
</html>
获取图片信息
传递文件对象获取
js
function getImageDetails(imageFile) {
return new Promise((resolve, reject) => {
// 检查是否为有效的图片文件
if (!imageFile || !imageFile.type.startsWith('image/')) {
reject('无效的图片文件');
return;
}
const img = new Image();
img.src = URL.createObjectURL(imageFile);
img.onload = () => {
// 释放对创建的对象URL的引用
URL.revokeObjectURL(img.src);
resolve({
width: img.width,
height: img.height,
size: imageFile.size
});
};
img.onerror = (error) => {
URL.revokeObjectURL(img.src);
reject('图片加载失败:', error);
};
});
}
// 使用示例
document.querySelector('input[type="file"]').addEventListener('change', (event) => {
const file = event.target.files[0];
if (!file) {
console.log('没有选择任何文件');
return;
}
getImageDetails(file)
.then(details => {
console.log(`图片宽度: ${details.width}px, 图片高度: ${details.height}px, 文件大小: ${(details.size / 1024).toFixed(2)}KB`);
})
.catch(error => {
console.error(error);
});
});
传递图片url或者base64转成文件对象
js
/**
* 根据图片的URL或Base64字符串创建并返回一个File对象。
* @param {string} imageSrc - 图片的URL或Base64字符串。
* @param {string} [fileName='image.jpg'] - 可选参数,指定生成的File对象的名字。
* @returns {Promise<File>} 返回一个包含File对象的Promise。
*/
async function createFileFromImage(imageSrc, fileName = 'image.jpg') {
let blob;
if (isBase64(imageSrc)) {
// 处理Base64字符串
const base64String = imageSrc.split(',')[1]; // 移除data URL部分
const mime = imageSrc.split(';')[0].split(':')[1]; // 获取MIME类型
const byteString = atob(base64String); // 解码Base64字符串
const arrayBuffer = new ArrayBuffer(byteString.length);
const uint8Array = new Uint8Array(arrayBuffer);
for (let i = 0; i < byteString.length; i++) {
uint8Array[i] = byteString.charCodeAt(i);
}
blob = new Blob([uint8Array], { type: mime });
} else {
// 处理URL
try {
const response = await fetch(imageSrc);
if (!response.ok) {
throw new Error(`网络响应失败: ${response.statusText}`);
}
blob = await response.blob();
} catch (error) {
console.error('获取图片时出错:', error);
throw error;
}
}
// 创建 File 对象
return new File([blob], fileName, { type: blob.type });
}
/**
* 判断给定的字符串是否为Base64编码的图片数据。
* @param {string} str - 要检查的字符串。
* @returns {boolean} 如果是Base64图片则返回true,否则返回false。
*/
function isBase64(str) {
const regexBase64 = /^data:image\/(png|jpg|jpeg|gif|bmp);base64,/;
return regexBase64.test(str);
}
// 使用示例
const imageUrl = 'https://example.com/path/to/your/image.jpg'; // 替换为你的图片链接
// 或者使用 Base64 字符串
// const imageBase64 = 'data:image/png;base64,iVBORw0KGgoAAAANSUhEUg...';
createFileFromImage(imageUrl, 'myImageFile.jpg')
.then(file => {
console.log('File对象创建成功:', file);
// 这里可以对file对象进行进一步操作
})
.catch(error => {
console.error(error);
});
传递图片url或者base64转成Image对象
js
/**
* 根据提供的图片URL或Base64字符串生成并返回一个图片对象。
* @param {string} imageUrlOrBase64 - 图片的URL或Base64字符串。
* @returns {Promise<HTMLImageElement>} 返回一个包含加载完成的图片对象的Promise。
*/
function createImage(imageUrlOrBase64) {
return new Promise((resolve, reject) => {
const img = new Image();
img.src = imageUrlOrBase64;
img.onload = () => {
resolve(img);
};
img.onerror = (error) => {
reject('图片加载失败: ' + error);
};
});
}
图片文件转换为Base64
js
/**
* 将图片文件转换为Base64字符串。
* @param {File} file - 通过文件输入控件获取的图片文件对象。
* @returns {Promise<string>} 返回一个解析为Base64字符串的Promise对象。
*/
function imageToBase64(file) {
return new Promise((resolve, reject) => {
const reader = new FileReader();
reader.readAsDataURL(file);
reader.onload = () => resolve(reader.result);
reader.onerror = error => reject(error);
});
}
// 使用示例
document.querySelector('input[type="file"]').addEventListener('change', async (event) => {
const file = event.target.files[0]; // 获取用户选择的第一个文件
if (!file) {
console.log('没有选择任何文件');
return;
}
try {
const base64String = await imageToBase64(file);
console.log('图片的Base64编码:', base64String);
// 可以在这里对base64String进行进一步处理,例如显示在页面上或上传到服务器
} catch (error) {
console.error('图片转换Base64失败:', error);
}
});
单位格式化工具
js
/**
* 根据文件的字节大小转换为合适的单位表示,并根据需要去除不必要的小数点和零。
* @param {number} bytes - 文件的字节大小。
* @returns {string} 返回带有合适单位的文件大小字符串。
*/
function formatFileSize(bytes) {
if (bytes === 0) return '0 B';
const k = 1024;
const sizes = ['B', 'KB', 'MB', 'GB', 'TB'];
const i = Math.floor(Math.log(bytes) / Math.log(k));
const formattedSize = bytes / Math.pow(k, i);
// 判断是否有小数部分,如果没有,则返回整数形式;如果有,则保留两位小数
return (formattedSize % 1 === 0 ? formattedSize.toFixed(0) : formattedSize.toFixed(2)) + ' ' + sizes[i];
}
// 使用示例
console.log(formatFileSize(1024)); // 输出: "1 KB"
console.log(formatFileSize(1536)); // 输出: "1.50 KB"
console.log(formatFileSize(2048)); // 输出: "2 KB"
console.log(formatFileSize(1048576)); // 输出: "1 MB"
console.log(formatFileSize(134217728)); // 输出: "128 MB"
文件转blob
传一个文件对象,返回一个blob数据,也就是一个临时的url
js
const img = new Image();
//blob:http://localhost:3002/bc31e37b-08fe-49b0-8201-61a6866ca8d8
console.log(URL.createObjectURL(imageFile))
img.src = URL.createObjectURL(imageFile);
img.onload = () => {
// 释放对创建的对象URL的引用
URL.revokeObjectURL(img.src);
};
img.onerror = (error) => {
URL.revokeObjectURL(img.src);
reject('图片加载失败:', error);
};