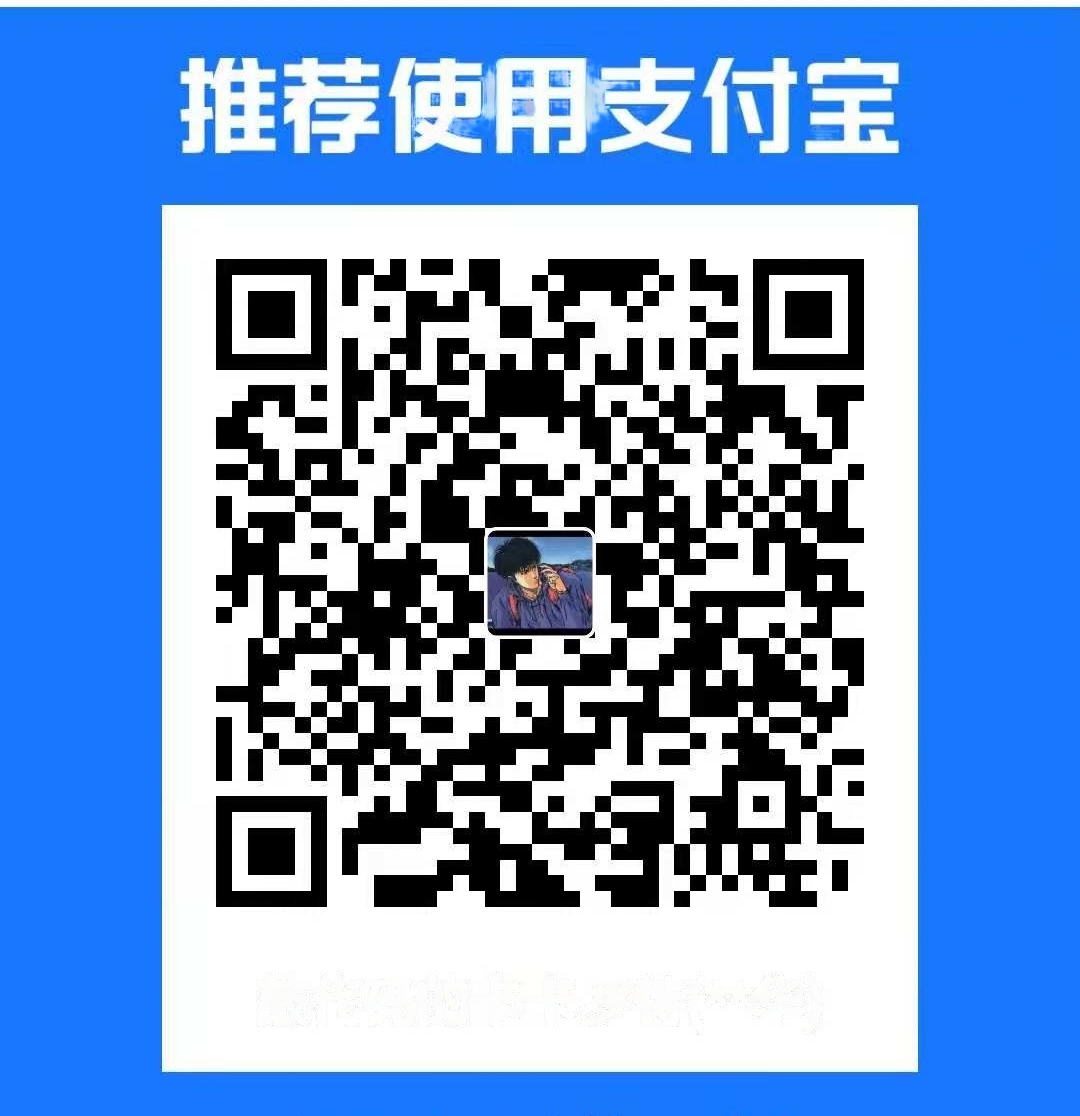
09-浏览器相关
windows事件
js
// 监听屏幕尺寸
window.onresize = function () {
}
// 页面加载完成后触发
window.onload = function() {
}
监听滚动条是否到达底部
判断滚动位置:通过判断滚动位置与页面高度的差值来判断是否到达底部。当滚动位置与页面高度的差值小于等于一个阈值时,即可认为到达底部。
js
window.addEventListener('scroll', function() {
var scrollTop = document.documentElement.scrollTop || document.body.scrollTop;
var windowHeight = window.innerHeight || document.documentElement.clientHeight || document.body.clientHeight;
var documentHeight = Math.max(document.body.scrollHeight, document.documentElement.scrollHeight);
var threshold = 50; // 阈值,可以根据实际情况调整
if (documentHeight - scrollTop - windowHeight <= threshold) {
// 到达页面底部的逻辑处理
console.log('到达页面底部');
}
});
使用Intersection Observer API:Intersection Observer API是一种现代的浏览器API,可以用于监测元素是否进入或离开视窗。 可以创建一个Intersection Observer实例,观察页面底部元素是否进入视窗,从而判断是否到达页面底部。
js
var options = {
root: null, // 默认为视窗
rootMargin: '0px',
threshold: 1.0 // 元素完全进入视窗时触发回调
};
var observer = new IntersectionObserver(function(entries, observer) {
entries.forEach(function(entry) {
if (entry.isIntersecting) {
// 到达页面底部的逻辑处理
console.log('到达页面底部');
}
});
}, options);
var target = document.querySelector('#bottom-element'); // 页面底部元素的选择器
observer.observe(target);
判断页面是否有滚动条
js
function hasScrollbar(element) {
if (element){ //判断某个元素是否有滚动条
return element.scrollHeight > element.clientHeight;
}else{ // 判断页面是否有滚动条
return document.body.scrollHeight > (window.innerHeight || document.documentElement.clientHeight);
}
}
判断是否是移动端
- 手机:通常认为小于 768px 的屏幕宽度属于手机。
- 平板:通常认为屏幕宽度在 768px 至 1024px 之间属于平板。
- 桌面:通常认为大于 1024px 的屏幕宽度属于桌面。
js
function detectDeviceType() {
const mobileThreshold = 768;
const tabletThreshold = 1024;
const screenWidth = window.innerWidth;
if (screenWidth >= tabletThreshold) {
return 'desktop';
} else if (screenWidth >= mobileThreshold && screenWidth < tabletThreshold) {
return 'tablet';
} else {
return 'mobile';
}
}
// 使用示例
const deviceType = detectDeviceType();
console.log(`Detected device type: ${deviceType}`);
onload到底是资源加载完成还是渲染完成
html
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<script>
alert('first');
</script>
<body >
<img class="currentImg" onload="alert('img1')" src="https://fastly.picsum.photos/id/67/200/130.jpg?hmac=24m0vrmpltCMoM7x_b5e_nyZAUIB6rqj6k37Gk3vgAo" alt=""/>
<img class="currentImg" onload="alert('img2')" src="https://fastly.picsum.photos/id/264/200/330.jpg?hmac=btS6pNync9pgxdFoBOhvIGRhREuIR-kZC3Gi4I7t1GQ" alt=""/>
</body>
<script>
window.onload = function(){
alert('last')
}
</script>
</html>
原理: 利用alert阻断浏览器渲染
结果:
1、输出依次是 first、img2、img1、last, 竟然先加载了第二个图片,说明资源加载时的
2、浏览器弹屏img2、img1时图片并未展示,说明onload调用时机是
3、去掉两个<img>
中的onload属性发现弹屏“last”时 ,即window.onload表示资源加载而非渲染结束后调用
4、将<img>
中的URL改成错误的URL发现window.onload同样调用了,但此时发现浏览器控制台已经输出了图片加载失败的error,
5、将两个<img>
中的URL都改成错误的URL,发现window.onload同样调用了,
浏览器注入外部JS文件
需要注意的是,这个js文件不能使用本地文件地址,使用网络路径。比如http://127.0.0.1/test.js
js
const createScript = document.createElement("script")
createScript.setAttribute("src", "远程js路径")
const doc = document.documentElement;
doc.insertBefore(createScript, doc.firstChild)
禁止右键操作
js
//禁止页面选择以及鼠标右键
document.οncοntextmenu = function () {
return false;
};
document.onselectstart = function () {
return false;
};
//禁用右键
document.oncontextmenu = function () {
return false;
};
//在本网页的任何键盘敲击事件都是无效操作 (防止F12和shift+ctrl+i调起开发者工具)
window.onkeydown = window.onkeyup = window.onkeypress = function () {
window.event.returnValue = false;
return false;
}
//禁用开发者工具F12
document.onkeydown = function () {
if (window.event && window.event.keyCode == 123) {
event.keyCode = 0;
event.returnValue = false;
return false;
}
};
url地址得到网站地址
js
function getWebsiteUrl(urlString) {
try {
// 使用URL对象解析url字符串
const url = new URL(urlString);
// 获取协议(http:或https:等)
const protocol = url.protocol;
// 获取主机名(例如 www.example.com)
const hostname = url.hostname;
// 获取端口号,默认是空字符串如果没有指定
const port = url.port ? `:${url.port}` : '';
// 构造网站地址
const websiteUrl = `${protocol}//${hostname}${port}`;
return websiteUrl;
} catch (error) {
// 如果输入的url无效,则返回错误信息
return 'Invalid URL';
}
}
// 示例使用
console.log(getWebsiteUrl('http://www.example.com/path/to/page?name=value&another=value'));
// 输出:http://www.example.com
console.log(getWebsiteUrl('https://sub.domain.com:8080/some/path'));
// 输出:https://sub.domain.com:8080
浏览器增加键盘事件
js
document.addEventListener('keydown', function(event) {
// 检查是否同时按下了Ctrl键和C键
if (event.ctrlKey && event.key === 'c') {
event.preventDefault(); // 可选,防止默认的复制操作
console.log('Ctrl+C 被按下');
// 在这里可以添加你的处理逻辑
const pasteText = window.getSelection().toString();
if (null === pasteText || undefined === pasteText || '' === pasteText.trim()) {
return;
}
navigator.clipboard.writeText(pasteText).then(() => {
alert("复制成功!");
}).catch(() => {
alert("复制失败!");
});
}
});
判断当前环境是生产还是开发
js
class DevUtil {
// 声明环境变量
static dev;
/**
* 获取当前环境(开发或生产)
* @returns {string} 'dev' 或 'prod'
*/
static getCurrentEnv() {
// 如果没有设置环境变量,则根据URL判断
if (!DevUtil.dev) {
const url = window.location.href;
const hostname = window.location.hostname;
// 检查是否为本地主机
if (hostname === 'localhost' || hostname === '127.0.0.1') {
DevUtil.dev = 'dev';
} else {
DevUtil.dev = 'prod';
}
}
return DevUtil.dev;
}
}
// 使用示例
console.log(DevUtil.getCurrentEnv()); // 输出 'dev' 或 'prod'