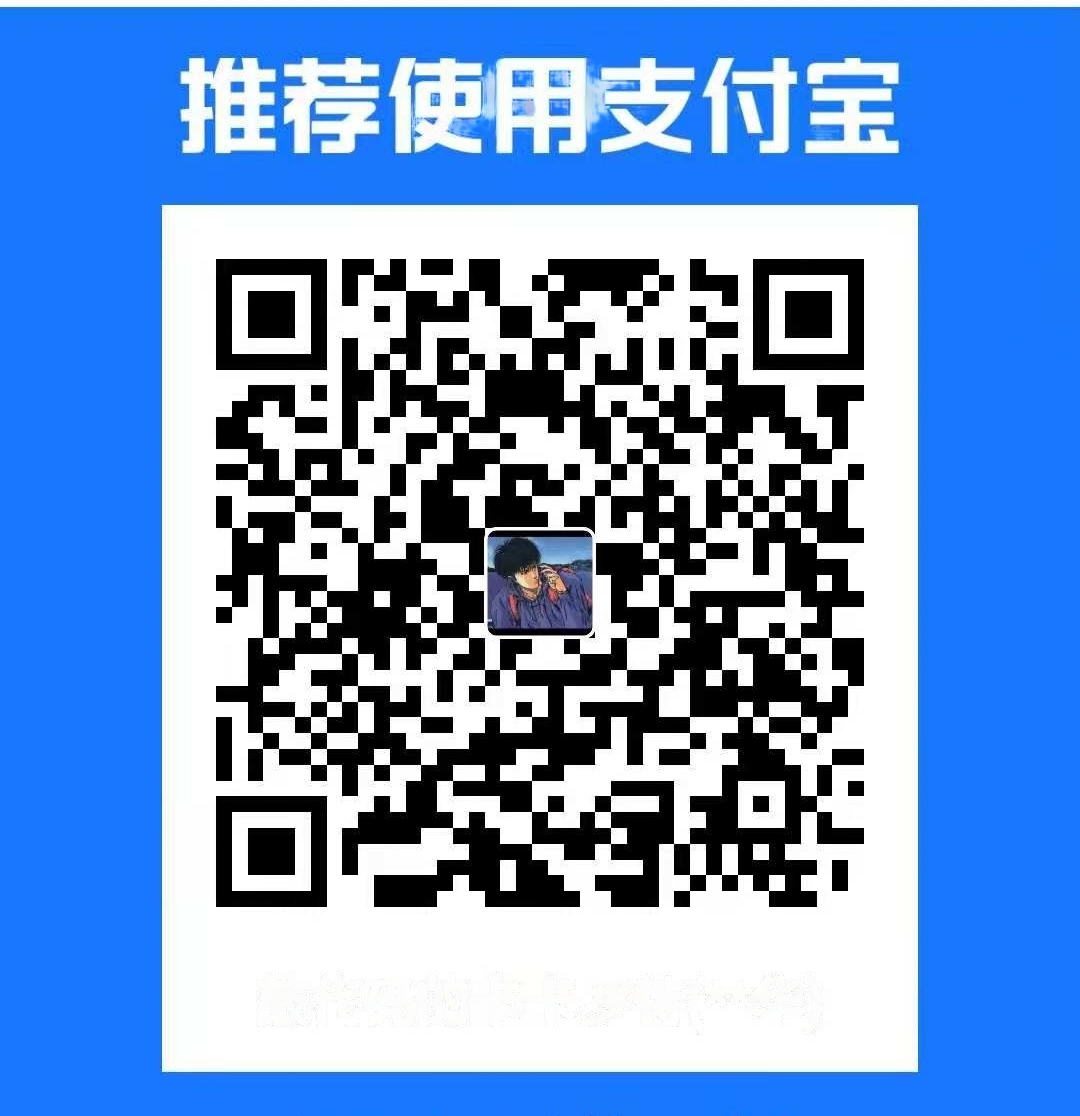
08-js数组相关
判断变量是否是对象
在 JavaScript 中,判断一个变量是否为对象有几种方法。下面是一些常见的方法:
方法一:使用 typeof 操作符
typeof 可以用来检测变量的基本类型,但是对于对象类型的变量(除了 null),它都会返回 "object"。
js
function isObject(obj) {
return typeof obj === 'object' && obj !== null;
}
console.log(isObject({})); // true
console.log(isObject(null)); // false
console.log(isObject([])); // true, 数组也是对象
console.log(isObject(42)); // false
方法二:使用 constructor 属性
检查对象的 constructor 属性是否指向 Object 构造函数。
js
function isObject(obj) {
return obj.constructor === Object;
}
console.log(isObject({})); // true
console.log(isObject([])); // false
方法三:使用 instanceof 关键字
instanceof 关键字用于测试构造函数的 prototype 属性是否出现在某个实例对象的原型链上。
js
function isObject(obj) {
return obj instanceof Object;
}
console.log(isObject({})); // true
console.log(isObject([])); // true
console.log(isObject(function () {})); // true, 函数也是对象
方法四:使用 toString 方法
Object.prototype.toString.call()
可以更精确地检测类型,因为它会返回一个表示对象类型的字符串。
js
function isPlainObject(obj) {
return Object.prototype.toString.call(obj) === '[object Object]';
}
console.log(isPlainObject({})); // true
console.log(isPlainObject([])); // false
console.log(isPlainObject(new Date())); // false
方法五:综合方法
结合 typeof 和 toString 方法来判断一个“纯”对象(即普通的 {} 对象):
js
function isPlainObject(obj) {
if (typeof obj !== 'object' || obj === null) return false;
let proto = obj;
while (Object.getPrototypeOf(proto) !== null) {
proto = Object.getPrototypeOf(proto);
}
return Object.getPrototypeOf(obj) === proto;
}
console.log(isPlainObject({})); // true
console.log(isPlainObject([])); // false
console.log(isPlainObject(new Date())); // false
这种方法可以更准确地判断是否是一个普通的对象,而不是数组或其他特殊对象。
根据你的具体需求选择合适的方法。如果你只是想确认是否是一个普通对象,推荐使用 isPlainObject 方法。如果你关心的是是否属于 Object 类型,而不排除其他特殊对象(如数组),可以选择使用 isObject 方法。
数组
forEach
js
const fruits = ['apple', 'banana', 'cherry'];
fruits.forEach((fruit, index) => {
console.log(`${index}: ${fruit}`);
});
// 输出:
// 0: apple
// 1: banana
// 2: cherry
数组转Map
js
const list = [
{ url: "aaa", lastmod: "xxx" },
{ url: "bbb", lastmod: "yyy" },
{ url: "ccc", lastmod: "zzz" }
];
const map = list.reduce((acc, item) => {
acc[item.url] = item.lastmod;
return acc;
}, {});
console.log(map);
// {
// aaa: 'xxx',
// bbb: 'yyy',
// ccc: 'zzz'
// }
数组去重
js
const arr = [1, 2, 2, 3, 4, 4];
const uniqueArr = Array.from(new Set(arr));
console.log(uniqueArr); // [1, 2, 3, 4]
数组过滤组装返回新的数组
js
const arr = [
{ name: 'Alice', url: 'aaa', lastmod: 'xxx' },
{ name: null, url: 'bbb', lastmod: 'yyy' },
{ name: 'Bob', url: 'ccc', lastmod: 'zzz' },
{ name: 'Charlie', url: 'ddd', lastmod: 'www' },
{ name: null, url: 'eee', lastmod: 'vvv' }
];
// 先过滤掉 name 为 null 的对象、再重新组装成新的数组,只保留 url 和 lastmod 字段
const transformedArr = arr.filter(item => item.name !== null).map(item => {
return {
url: item.url,
lastmod: item.lastmod
}
});
console.log(transformedArr);
// [
// { url: 'aaa', lastmod: 'xxx' },
// { url: 'ccc', lastmod: 'zzz' },
// { url: 'ddd', lastmod: 'www' }
// ]
数组排序
js
// sort方法有两个注意点:
//
// 会操作原始数组,经过操作后原始数组发生变化
// 默认排序按照字符编码排序,例如,我们有下面的一个例子:
var arr1 = [14,23,11,6,87,67];
arr1.sort(); // [11,14,23,6,67,87] 按字符而非数值排序
// 想要完成值比较排序,必须传入sort参数(函数)进行规制制定:
// 从小到大排序 [6, 11, 14, 23, 67, 87]
arr1.sort((a,b) => a - b );
提取字段成新数组
js
let b = arr.map(x => {return x.imgUrl})
数组截取
slice是截取索引a到索引b,[a,b) 左闭右开的原则
js
let array = [1,2,3,4,5];
// 确保不超过数组的实际长度
let first20Items = array.slice(0, Math.min(array.length, 3));
console.log(first20Items); //[1, 2, 3]
对象
对象拷贝
js
const BeanUtil = {
/**
* 只拷贝目标对象字段
* 两个对象属性值copy
* @param source
* @param target
*/
copy(source,target){
// 简写形式: Object.keys(target).forEach(k => k in source && (target[k] = source[k]));
if (source && target){
for (let key in source) {
if (target.hasOwnProperty(key)) {
target[key] = source[key];
}
}
}
}
};
export default BeanUtil
遍历对象
js
for (let key in obj) {
if (obj.hasOwnProperty(key)) {
console.log(key + ": " + obj[key]);
}
}
对象的键转换为数组
js
const a = { name: 'aa',age: '18' };
const arr = Object.keys(a);
console.log(arr); // 输出 [name, age]
将对象的值转换为数组
js
const a = { name: 'aa',age: '18' };
const arr = Object.values(a);
console.log(arr); // 输出 [aa, 18]
将对象的键值对转换为数组的元素
js
let a = {name: 'aa',age: '18'}
const arr = Object.entries(a).map(([key, value]) => [key, value]);
console.log(arr, 'arr'); // [["name","aa"],["age","18"]]