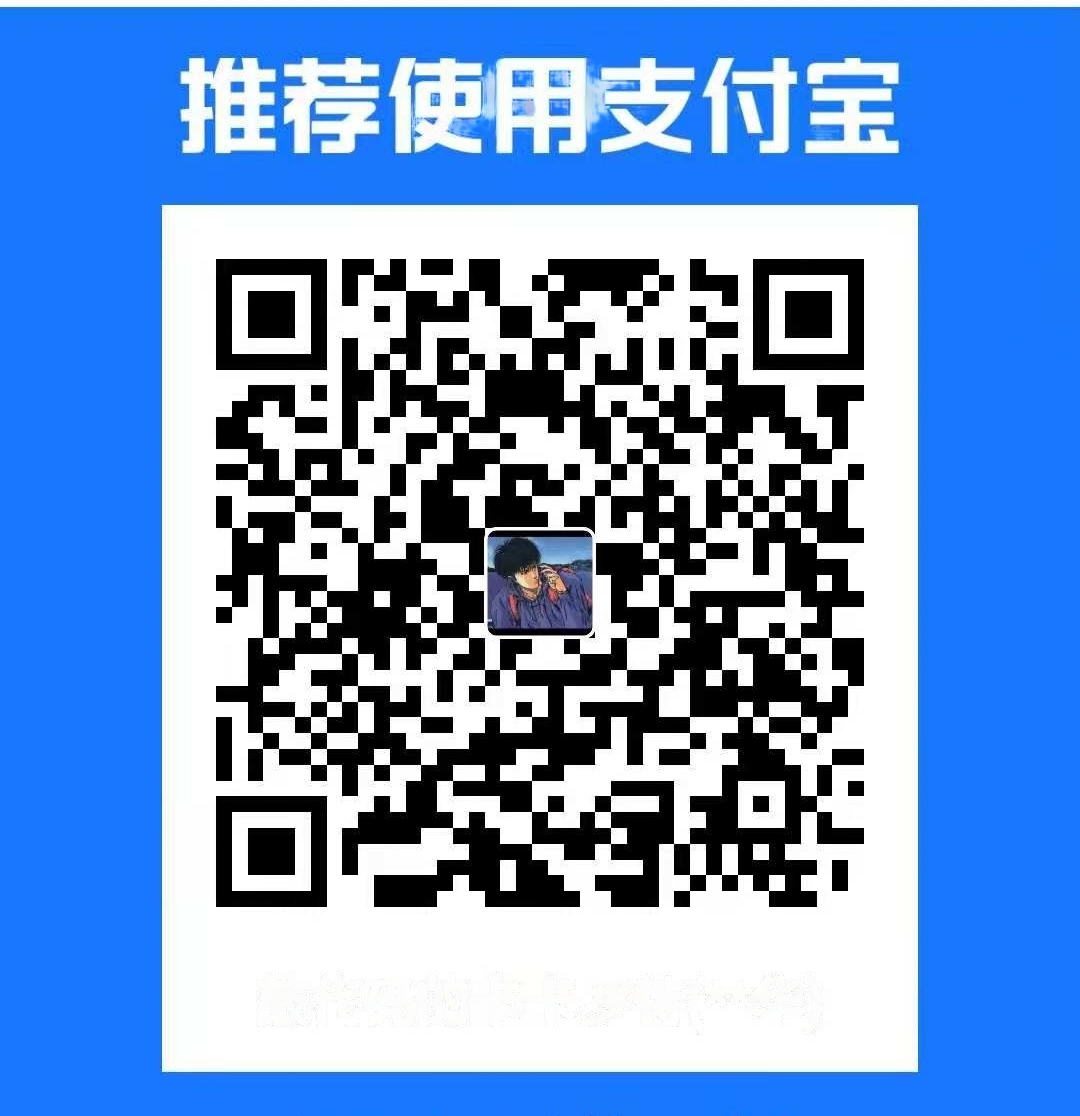
11-DOM相关
获取元素
closest()方法可以沿着DOM树向上遍历,直到找到匹配的选择器的第一个祖先元素(包括它自己)。然后你可以从这个祖先元素中选择你想要的子元素。
js
// 从按钮开始,找到最近的.item容器
let itemContainer = document.getElementById('myId').closest('.item');
js
// 在.item容器内查找img元素
let imgElement = itemContainer.querySelector('.item-img img');
DOM插入子元素
使用 innerHTML
这是最简单的方法之一,但需要注意这会替换元素中的所有内容。
js
const element = document.getElementById('myElement');
element.innerHTML += '<div>这是一个新的片段</div>';
使用insertAdjacentHTML
这个方法允许你更灵活地插入 HTML 片段而不替换元素中已有的内容。
js
const element = document.getElementById('myElement');
element.insertAdjacentHTML('beforeend', '<div>这是一个新的片段</div>');
在 insertAdjacentHTML 方法中,第二个参数指定了如何插入新内容。可用的选项包括:
- beforebegin: 在元素之前
- afterbegin: 在元素内部的最开始
- beforeend: 在元素内部的最后
- afterend: 在元素之后
html
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>DOM 示例</title>
</head>
<body>
<div id="myElement">
<p>原始内容</p >
</div>
<button id="addContent">添加内容</button>
<script>
document.getElementById('addContent').addEventListener('click', () => {
// 使用 insertAdjacentHTML 添加内容
const element = document.getElementById('myElement');
element.insertAdjacentHTML('beforeend', '<div>这是一个新的片段</div>');
});
</script>
</body>
</html>
使用appendChild
如果你想创建元素并添加事件,也可以使用 appendChild 方法:
js
const element = document.getElementById('myElement');
const newDiv = document.createElement('div');
newDiv.textContent = '这是一个新的片段';
element.appendChild(newDiv);
字符串转DOM
js
function parseHTML(htmlString) {
const parser = new DOMParser();
const doc = parser.parseFromString(htmlString, 'text/html');
return doc.body.firstChild; // 返回第一个子元素
}
const htmlString = '<div><p>Hello, World!</p ></div>';
const domElement = parseHTML(htmlString);
console.log(domElement); // 输出: <div><p>Hello, World!</p ></div>